Archiving file attachments
If a conversation includes file attachments, e.g. images, documents, audio, etc., you must send the files to the /files endpoint before you reference them in the /conversations request.
For each attachment, make a PUT request to the /files endpoint, with your chosen fileKey included as a URL path parameter:
curl -X PUT "conversations.api.globalrelay.com/v2/files/default/documents/Q3-report-2023.pdf" \
-H "Authorization: Bearer <YOUR_ACCESS_TOKEN>" \
-H "Content-Type: <VALID_CONTENT_TYPE>" \
-T "<PATH_TO_FILE>"
If you use the same fileKey for multiple requests, the API returns a 200 success response, and the original file is replaced with the new version specified in the latest request.
You must include “/default” in the URL path before the file name. The path from “default” onward, e.g. “default/documents/Q3-report-2023.pdf”, will be the fileKey value you reference in the /files endpoint.
Handling different file types
The file should be sent as binary data in the body of the PUT request, and you should include a Content-Type header with a standard MIME type describing the format of the contents.
If the file type is unknown, you can use the generic “application/octet-stream” type; however, the file type will not be obvious to a user of the Archive and they may not be able to open the file.
A Content-Length header is not required; however, if you send an incorrect value, only the amount of data specified in the header will be captured, which could result in incomplete data being archived. As per RFC 9110, the Content-Length should be the uncompressed, decrypted file size as a decimal, non-negative integer number of octets.
The /files endpoint supports files up to 1.0GB in size. If you send a file larger than this, the API returns a 400 error.
Referencing attached files
Once you receive a 200 success response from the /files endpoint for all files referenced in the conversation, make a request to the /conversations endpoint referencing the files with the “File_transfer” eventType.
Additionally, you must include the user/system actor who shared the file and, if applicable, the targeted recipients.
It’s essential that the fileKey in this request matches the fileKey uploaded via the PUT /files endpoint.
If you supply a fileKey which is not found in your Archive, the POST /conversations request will return a 400 error response and the associated conversation data will not be archived.
For example, Jordan shared a file with all the conversation participants, so they don’t need to be explicitly declared:
{
"conversationEvents": [
{
"eventTime": 1621851918000,
"eventType": "File_transfer",
"participants": [
{
"displayName": "Jordan Davies",
"corporateEmail": "jordan.davies@greenvaultcapital.com",
"userType": "initiator"
}
],
"files": [
{
"filename": "Q3-report-2023.pdf",
"fileKey": "default/documents/Q3-report-2023.pdf",
"isInlined": false
}
]
}
]
}
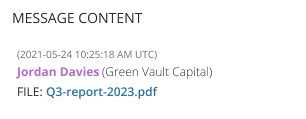
In this example, a single file was uploaded for the “File_transfer” event; however, you can include multiple files in the same event, e.g. if a user uploaded multiple images in a single instance.
Sending a file to specific users in a conversation
If a file is shared with specific users in a conversation, not all participants, you must declare those users in the list of ConversationEvent.participants.
For example, in a group chat with 10 users, Jordan shared his file with Lisa only. Therefore, you would identify Jordan as the “initiator” and Lisa as the “recipient”:
{
"conversationEvents": [
{
"eventTime": 1621851918000,
"eventType": "File_transfer",
"participants": [
{
"displayName": "Jordan Davies",
"corporateEmail": "jordan.davies@greenvaultcapital.com",
"userType": "initiator"
},
{
"displayName": "Lisa Holmes",
"corporateEmail": "lisa.holmes@greenvaultcapital.com",
"userType": "recipient"
}
],
"files": [
{
"filename": "Q3-report-2023.pdf",
"fileKey": "default/documents/Q3-report-2023.pdf",
"isInlined": false
}
]
}
]
}
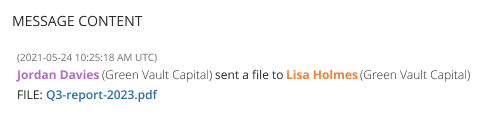
When referencing the file in your POST request to the Conversation Archiving API, include the file path from “default” onward.
For example, if you sent this request to the /files endpoint:
curl -X PUT "https://conversations.api.globalrelay.com/v2/files/default/documents/Q3-report-2023.pdf"
In the /conversations request, the path from “default” onward is the fileKey value:
"fileKey": "default/documents/Q3-report-2023.pdf"
Displaying shared images in the archived conversation
Control how shared images display in your archived conversations by using the files.isInlined field.
To display images alongside conversation text in the Archive, set this field to true for images you send in your API requests. If you set the field to false, which is the default, the images won’t display; however, they will be attached to the archived message with a hyperlink in the conversation body.
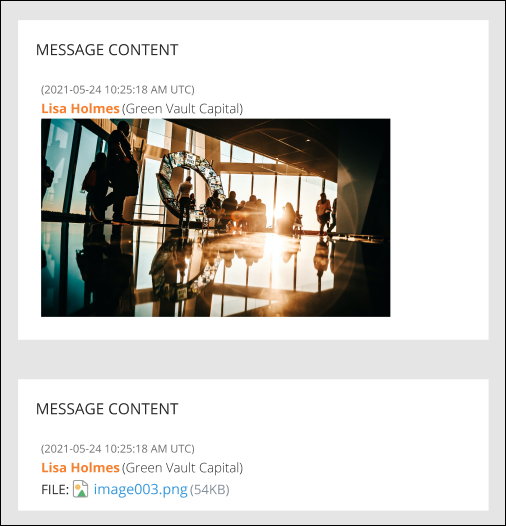
Other file types you share in conversations are always attached to the archived message via a hyperlink. Therefore, the files.isInlined field is not applicable for those file types.
Referencing external file locations
You can also include links to externally located files, which are not in your Global Relay Archive, by referencing the URL in the fileHref field along with the mandatory fileKey and filename fields.
When users view conversation data in your Archive, the filename will be the text displayed on the link to the URL you supply in fileHref. The fileKey is not displayed, but must be populated with some value in the API request.
Including message content with a file attachment
If a participant included message content with a file upload, you can specify it in the content object of the “File_transfer” event:
{
"conversationEvents": [
{
"eventTime": 1621851918000,
"eventType": "File_transfer",
"participants": [ // ],
"files": [ // ],
"content": {
"message": "take a look at these files"
}
}
]
}
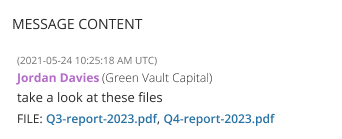
Accompanying file attachments with system text
For all Conversation Events, you can include accompanying text, which was shown to the participants of the conversation, by populating the systemText field.
{
"conversationEvents": [
{
"eventTime": 1621851918000,
"eventType": "File_transfer",
"participants": [ // ],
"files": [ // ],
"systemText": "User shared a file."
}
]
}
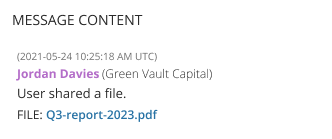